Lumen
Lumen was the first world the CODEX system successfully tunneled to. Although the scripted variables were simple, the world Professor Lauer visited was breathtaking.
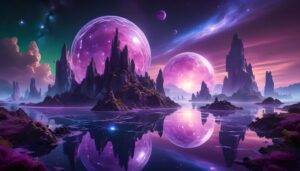
As I stepped through the open tunnel I had created just moments ago, I was instantly transported into this strange new world. The sight that greeted me was beyond any dream or fantasy I could have conjured. Towering crystal spires rose majestically from the ground, their sharp peaks piercing the sky, each one glowing with an ethereal light that mirrored the hues of the surrounding landscape. The terrain was a stunning expanse of iridescent colors, shifting and changing with every angle of the light.
The sky above was an enchanting deep purple, an endless canvas that stretched into the cosmos. Two gigantic, luminous orbs, for lack of a better word, hung in the heavens, their presence dominating the sky and casting a surreal glow over everything below. Their light created an otherworldly ambiance, reflecting off the crystal structures and the serene waters that bordered the spires.
The water itself was a deep, tranquil mirror, perfectly capturing the grandeur of the crystalline towers and the celestial bodies above. It was as if the entire world was caught in a perpetual state of twilight, a place where day and night blended seamlessly into one another.
Everywhere I looked, the scene was painted with rich, vivid purples, deep blues, and hints of green, creating a mesmerizing palette that was both calming and awe-inspiring. The reflections in the water added a layer of depth to the landscape, making it seem as though the world extended infinitely in all directions.
The air was filled with a sense of stillness and tranquility, the perfect silence only occasionally broken by the distant hum of the energy-based life forms I knew inhabited this realm. Their presence, though not immediately visible, could be felt as a subtle, harmonious vibration in the environment.
Excerpt from the journal of Professor Lauer
Lumen’s DEXscript
#!/usr/codex/env dexscript
import calc
import siglib
# Constants
EARTH_STANDARD = 1.0
# World properties
WORLD_NAME = "Lumen"
WORLD_DESC = "Crystalline Nebula"
GRAVITY = 0.3 * EARTH_STANDARD
PRIMARY_ELEMENT = "SILICON"
# Atmosphere properties
NITROGEN = 2.1
OXYGEN = 0.21
CARBON_DIOXIDE = 0.0004
ARGON = 0.9
TOTAL_PRESSURE = NITROGEN + OXYGEN + CARBON_DIOXIDE + ARGON
# Terrain properties
TERRAIN_TYPE = "OCEAN_ISLANDS"
TERRAIN_MATERIAL = "CRYSTAL"
TERRAIN_COLOR = "IRIDESCENT"
# Population properties
LIFEFORM = "ENERGY_BASED"
INTELLIGENCE = "HIVE_MIND"
INTERACTION = "NEUTRAL"
# Sky properties
SKY_COLOR = "DEEP_PURPLE"
SKY_FEATURE = "TWIN_SUNS"
# Safety check atmosphere
def is_atmosphere_safe():
safe = True
if NITROGEN > 3.0:
safe = False
if OXYGEN < 0.16 or OXYGEN > 0.5:
safe = False
if CARBON_DIOXIDE > 0.005:
safe = False
if ARGON > 1.6:
safe = False
return safe
# Safety check gravity
def is_gravity_safe(gravity):
# Safe range: 1% to 138% of Earth's gravity (inclusive)
return 0.01 <= gravity <= 1.38
# Calculate tunnel coordinates
def calculate_tunnel_coordinates(world_name, world_desc, gravity, primary_element, terrain_type, lifeform, intelligence, interaction, sky_color, sky_feature):
combined_input = (world_name + world_desc + primary_element + terrain_type + lifeform + intelligence + interaction + sky_color + sky_feature)
unique_signature = siglib.md5(combined_input.encode()).hexdigest()
large_int = int(unique_signature, 16)
coord_x = (large_int % 10000) / 1000.0 # X coordinate
coord_y = ((large_int // 10000) % 10000) / 1000.0 # Y coordinate
coord_z = ((large_int // 100000000) % 10000) / 1000.0 # Z coordinate
coord_x = calc.sin(coord_x) * 42.0
coord_y = calc.cos(coord_y) * 42.0
coord_z = calc.tan(coord_z) * 42.0
coord_x = (coord_x % 2000) - 1000
coord_y = (coord_y % 2000) - 1000
coord_z = (coord_z % 2000) - 1000
return (coord_x, coord_y, coord_z)
# Initialize world
def initialize_world():
atmosphere_safety = is_atmosphere_safe()
gravity_safety = is_gravity_safe(GRAVITY)
tunnel_coordinates = calculate_tunnel_coordinates(
WORLD_NAME, WORLD_DESC, GRAVITY, PRIMARY_ELEMENT, TERRAIN_TYPE, LIFEFORM, INTELLIGENCE, INTERACTION, SKY_COLOR, SKY_FEATURE
)
return atmosphere_safety, gravity_safety, tunnel_coordinates
initialize_world()